Our mission is to make it as easy as possible to go from idea to product, and this is something AI is gonna help us with.
So naturally, with the release of ChatGPT, we’ve been investigating how we could make use of it.
In this blogpost I’m focusing on how ChatGPT could help in development. I’m mostly a frontend guy, so I’ll be using it to prototype some simple web components.
Let’s go! To make clear what is being said by ChatGPT, I’ve marked its responses in purple.
“Create code for a web component that is responsible for displaying a table in html.”
Here is an example of how you could create a web component that displays a table in HTML:
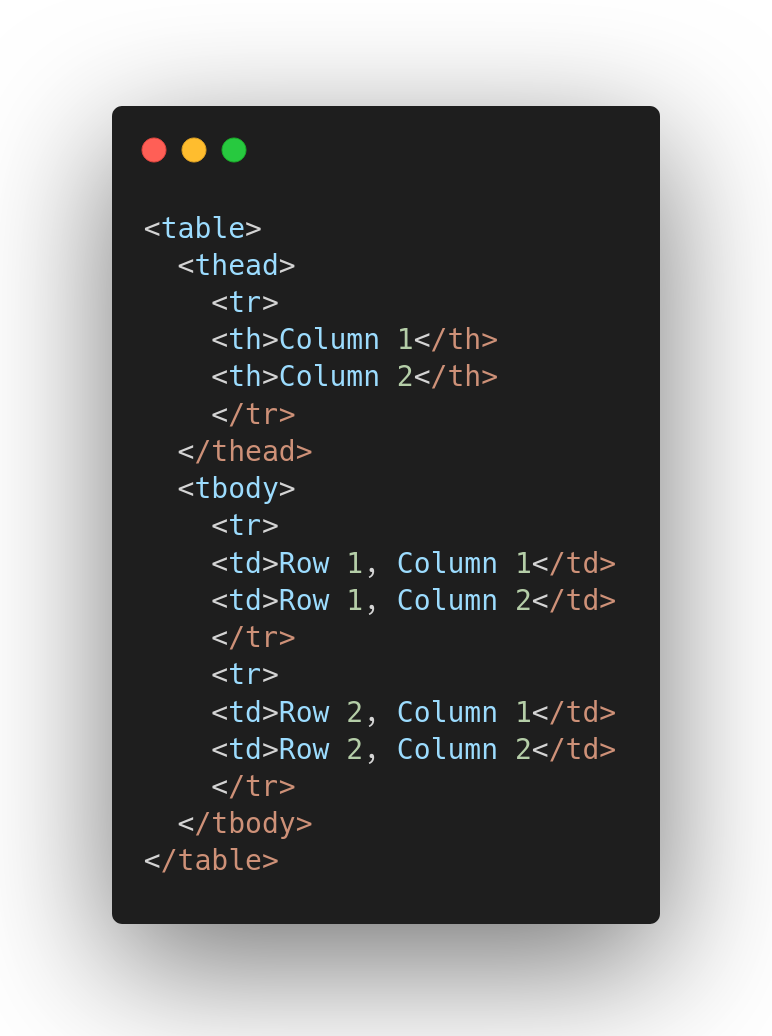
To create a web component, you would need to use a framework like React or Angular. Here is an example of how you could create a <table-component> using React:
You could then use this web component in your HTML like this:
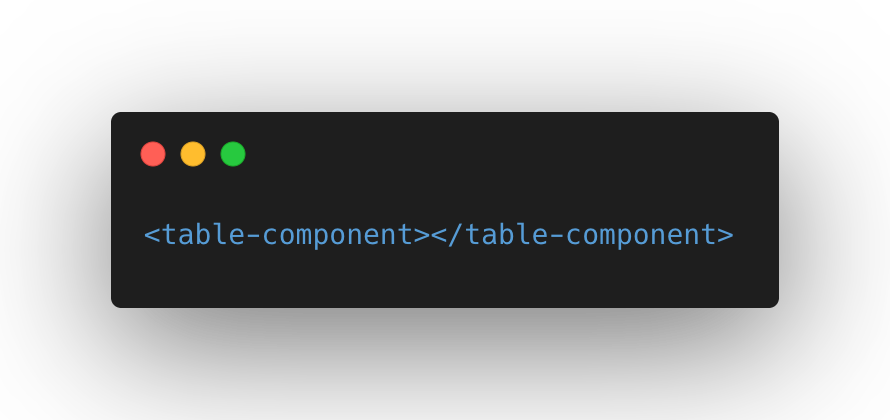
This is just a basic example to show how you could create a web component that displays a table in HTML. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
Pretty cool, but it’s not really a web component, is it. Let’s just try changing some words in the prompt, we might get lucky.
“Create javascript code for a web component that is responsible for displaying a table in html.”
Here is an example of how you could create a web component that displays a table in HTML using JavaScript:
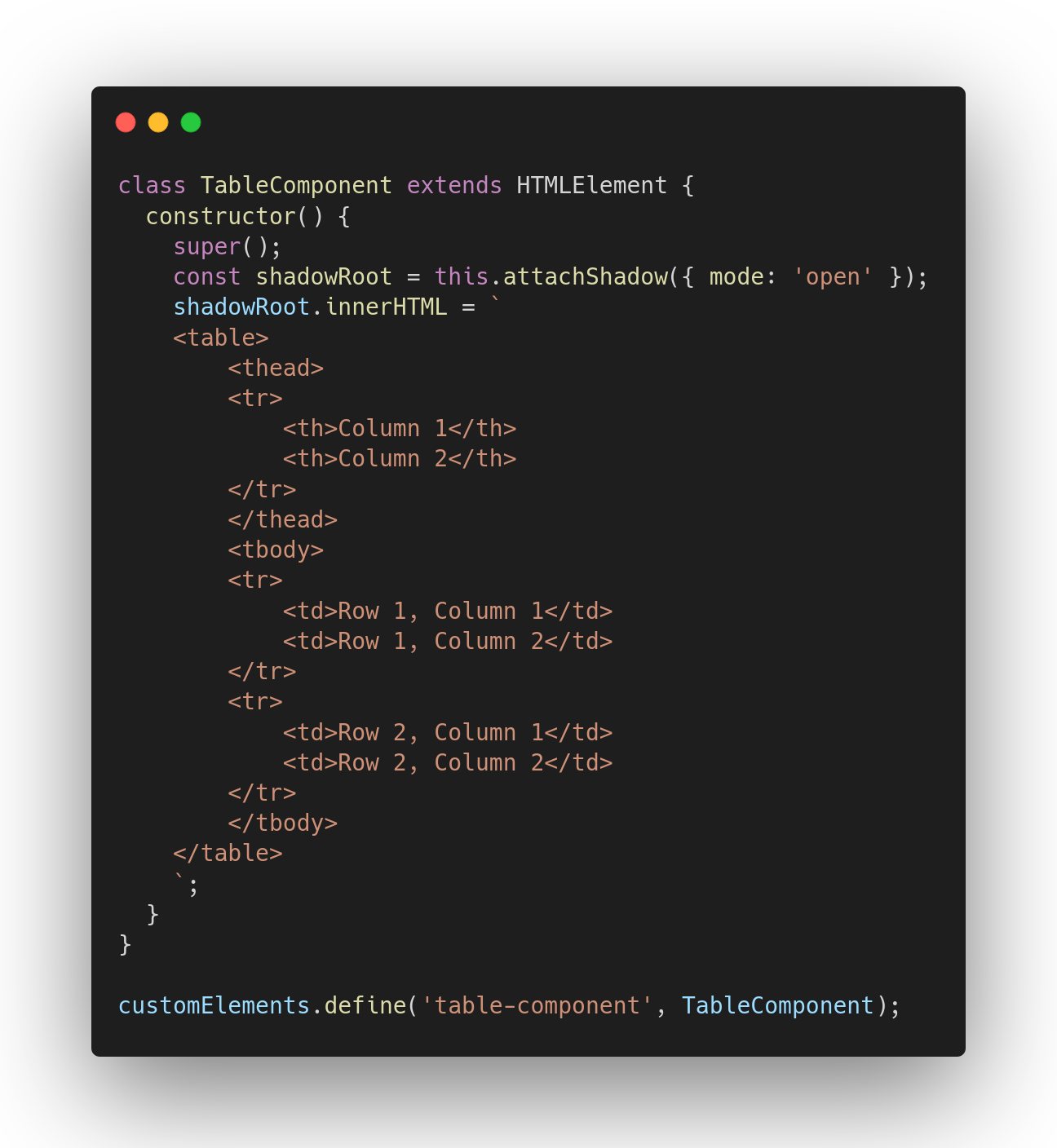
This is just a basic example to show how you could create a web component that displays a table in HTML using JavaScript. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
Yes! This is kind of what I’m looking for. It is even rendering the same table.
Now let’s try to adapt this code so that the table can be used to display different kinds of data.
“Can you alter the code of the component you just shared with me so that it reads the values of attributes to determine what needs to be presented in the table?”
Yes, you can use the getAttribute() method to read the values of attributes on the web component. For example, you could add two attributes to the web component, column-names and row-data, and use those to determine what needs to be displayed in the table.
Here is an example of how you could modify the code to read the values of these attributes:
You could then use this web component in your HTML like this, passing the values of the column-names and row-data attributes as JSON strings:
This is just one way that you could modify the web component to read the values of attributes and use those to determine what needs to be displayed in the table. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
Wow! This will actually work! I’m kind of impressed.
What is interesting is that it starts to explain how to use getAttribute. I guess that is because I started my prompt with “Can you”. However in this case I wasn’t implying that I didn’t know, I was just trying to be polite. You know, robot uprising and all that.
But from here on I’ll take on a more impersonal tone.
Usually when we need a more reactive component we typically use Lit – so let’s try to convert it.
“Rewrite the component you just shared to a lit component”
Here is an example of how you could rewrite the web component to a lit component:
You could then use this web component in your HTML like this, passing the values of the column-names and row-data attributes as JSON strings:
This is just one way that you could rewrite the web component to a lit component. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
Seems solid! However I actually wanted it to use a different library. As ChatGPT seems to be quite capable already, let’s just nudge it towards the right answer instead of fully explaining what it is that we want.
“I noticed that you used lit-element, please use the library from https://github.com/lit/lit/ instead”
Here is an example of how you could rewrite the web component to use the lit library from https://github.com/lit/lit/ instead of lit-element:
You could then use this web component in your HTML like this, passing the values of the column-names and row-data attributes as JSON strings:
This is just one way that you could rewrite the web component to use the lit library. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
It is getting more and more impressive. I’m starting to worry about my job here.
Personal stuff aside – it actually refactored the code quite nicely to make use of Lit. However it’s still not a typical lit-based component.
One of the things that is missing, is that it’s not yet extending LitElement, which is what would make this component reactive.
Q: Make the table extend LitElement instead, and make the properties reactive
Here is an example of how you could rewrite the web component to extend LitElement and make the properties reactive:
You could then use this web component in your HTML like this, passing the values of the column-names and row-data attributes as JSON strings:
This web component will now automatically update the table when the values of the column-names and row-data properties change. This is just one way that you could make the properties reactive. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
Yes! This kind of cleans things up a bit. I hoped it would actually also use the @property decorator to define the individual properties, but I guess this also gets the job done. But hey, let’s just ask it to make use of it anyway.
“Please define the properties through the @property decorator”
Here is an example of how you could use the @property decorator to define the properties of the web component:
You could then use this web component in your HTML like this, passing the values of the column-names and row-data attributes as JSON strings:
This web component will now automatically update the table when the values of the column-names and row-data properties change. This is just one way that you could use the @property decorator to define the properties of the web component. There are many different ways to implement this, and you may need to modify the code depending on your specific requirements.
Human reaction
At this point I finally noticed that ChatGPT made a small mistake – the attributes shown in the example code won’t work in this component.
Can ChatGPT figure out its own mistake?
“You made a small mistake – the attribute names that you provided in your example won’t be picked up by the web component. Can you guess the problem and fix it?”
The problem is that the attribute names that were used in the HTML example are not the same as the property names defined in the web component. In the web component, the property names are columnNames and rowData, but in the HTML example, the attribute names are column-names and row-data.
To fix this, you can either change the property names in the web component to use dashed names (e.g. column-names and row-data), or you can change the attribute names in the HTML example to use camel case (e.g. columnNames and rowData).
Here is an example of how you could fix this by changing the attribute names in the HTML example to use camel case:
You could then use this web component in your HTML like this, using camel case attribute names:
This should fix the problem and allow the web component to correctly read the values of the attributes. You may need to modify the code depending on your specific requirements.
Human reaction
This is beyond impressive. AI will truly rule us one day. Until that point, we can use them as our assistants.
Actually this is still a simple web component, but the fact that I can actually go through an iterative process of refining makes me feel like I’m actually working together with someone.
Let’s see how much further we can go!
“Extend the component so that the data is searchable through an input in the right-top of the rendered html”
To add a search input to the web component, you can add an input element to the HTML and bind its value to a property on the component. You can then use this property to filter the rows of the table.
Here is an example of how you could extend the web component to include a search input and filter the rows of the table:
You could then use this web component in your HTML like this, using camel case attribute names:
Human reaction
At this point ChatGPT was probably getting overloaded, which is probably why I didn’t get a full answer. Because I got what I needed – I’ll just continue with the next prompt. Let’s do some more cleanup!
“Please split up the render function in logical parts”
To split up the render function into logical parts, you can create separate functions for each part of the rendering. For example, you could create a function to render the search input, a function to render the table header, and a function to render the table body.
Here is an example of how you could split up the render function into separate functions:
This web component will now split the render function into separate functions for rendering the search input, the table header, and the table body. This makes the code more organized and easier to read. You may need to modify the code depending
Human reaction
Cut off again – but I must say I’m super impressed with this technology. It actually cleaned up the code quite nicely. I’ll stop with my prompts because ChatGPT seems to be overloaded.
Conclusion
It is insane how far we can go already.
At this point ChatGPT is already usable enough to lay the groundwork for some simple components.
Working with it actually feels nice too. You can just spew thoughts to your robotic colleague, who will then convert these thoughts into actual functioning code.
It is already proving to be useful, and we can only expect it to become even more advanced in the future.
Through Stekz and the power of AI, users don’t have to understand tons of technologies before they are able to end up with a software product that does exactly what they want.
AI will close the gap between business and the technical domain, and this is happening even faster than we predicted.
You are actually a excellent webmaster. This web site loading velocity is amazing.
It sort of feels that you are doing any distinctive
trick. In addition, the contents are masterpiece.
you’ve performed a fantastic activity on this matter!
Similar here: najlepszy sklep and also here: Bezpieczne zakupy